uni-app技术分享| 10分钟实现一个简易uniapp视频通话
视频讲解
创建 uniapp 项目
引入插件
云打包购买插件(免费引入)引入创建的对应uniapp 项目
uniapp项目的 中 选择云端插件anyRTC音视频SDK插件`
打包自定义基座
选择自定义基座运行
代码逻辑
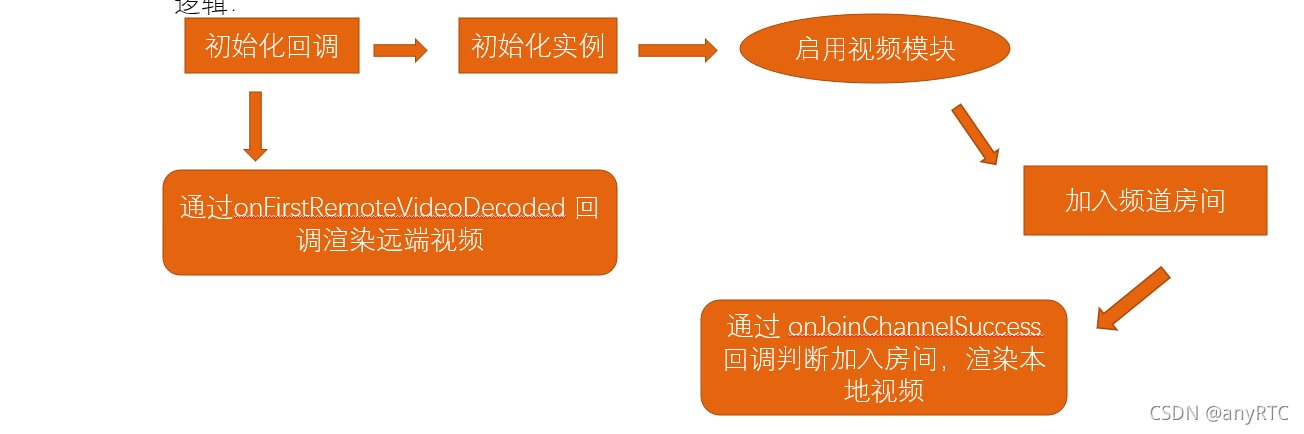
必须在 页面中
本地用户 {{localuid}} 音视频渲染
远端 {{remotenableuid}} 音视频渲染
// rtc 音视频引入
const rtcModule = uni.requireNativePlugin('AR-RtcModule');
export default {
data() {
return {
appid: "177e21c0d1641291c34e46e1198bd49a",
channel: "123456",
localuid: "", // 本地用户
remotenableuid: "", // 远端用户
}
},
onLoad() {
},
methods: {
// 步骤一:
stepOne() {
rtcModule.setCallBack(res => {
switch (res.engineEvent) {
// 发生警告回调
case "onWarning":
console.log("发生警告回调", res);
break;
// 发生错误回调
case "onError":
console.log("发生错误回调", res);
break;
// 加入频道成功回调
case "onJoinChannelSuccess":
console.log("加入频道成功回调", res);
// 本地用户视频渲染
this.localVideo();
break;
// 远端用户加入当前频道回调
case "onUserJoined":
uni.showToast({
title: '用户' + res.uid + '加入频道',
icon: 'none',
duration: 2000
});
break;
// 远端用户离开当前频道回调
case "onUserOffline":
uni.showToast({
title: '远端用户' + res.uid + '离开频道',
icon: 'none',
duration: 2000
});
break;
// 已显示远端视频首帧回调
case "onFirstRemoteVideoDecoded":
console.log("已显示远端视频首帧回调", res);
// 远端视频渲染
this.remotenableVideo(res.uid);
break;
// 远端用户视频状态发生已变化回调
case "onRemoteVideoStateChanged":
console.log("远端用户视频状态发生已变化回调", res);
break;
}
});
},
// 步骤二:
stepTwo() {
rtcModule.create({
"appId": this.appid
}, res => {
console.log('初始化实例 rtc', res);
});
// 智能降噪
// rtcModule.setParameters({
// Cmd: 'SetAudioAiNoise',
// Enable: 1,
// }, (res) => {
// console.log('私人定制', res);
// });
},
// 步骤三:
stepThree() {
rtcModule.enableVideo((res) => {
console.log('RTC 启用视频 enableVideo 方法调用', (res.code === 0 ? '成功' : '失败:') +
res);
});
},
// 步骤四:
stepFour() {
this.localuid = this.randomFn(6);
rtcModule.joinChannel({
"token": "",
"channelId": this.channel,
"uid": this.localuid,
}, (res) => {
console.log('RTC joinChannel 方法调用', (res.code === 0 ? '成功' : '失败:') + res);
});
},
// 本地视频渲染
async localVideo() {
// 渲染视频
await this.$refs.location.setupLocalVideo({
"renderMode": 1,
"channelId": this.channel,
"uid": this.localuid,
"mirrorMode": 0
}, (res) => {
console.log('渲染视频', res);
});
// 本地预览
await this.$refs.location.startPreview((res) => {
console.log('本地预览', res);
})
},
async remotenableVideo(uid) {
this.remotenableuid = uid;
this.$refs.remotenable.setupRemoteVideo({
"renderMode": 1,
"channelId": this.channel,
"uid": uid,
"mirrorMode": 0
}, (res) => {
console.log('渲染视频', res);
});
},
// 随机生成
randomFn(len, charSet) {
charSet = charSet || 'abcdefghijklmnopqrstuvwxyz0123456789';
let randomString = '';
for (let i = 0; i < len; i++) {
let randomPoz = Math.floor(Math.random() * charSet.length);
randomString += charSet.substring(randomPoz, randomPoz + 1);
}
return randomString;
},
}
}
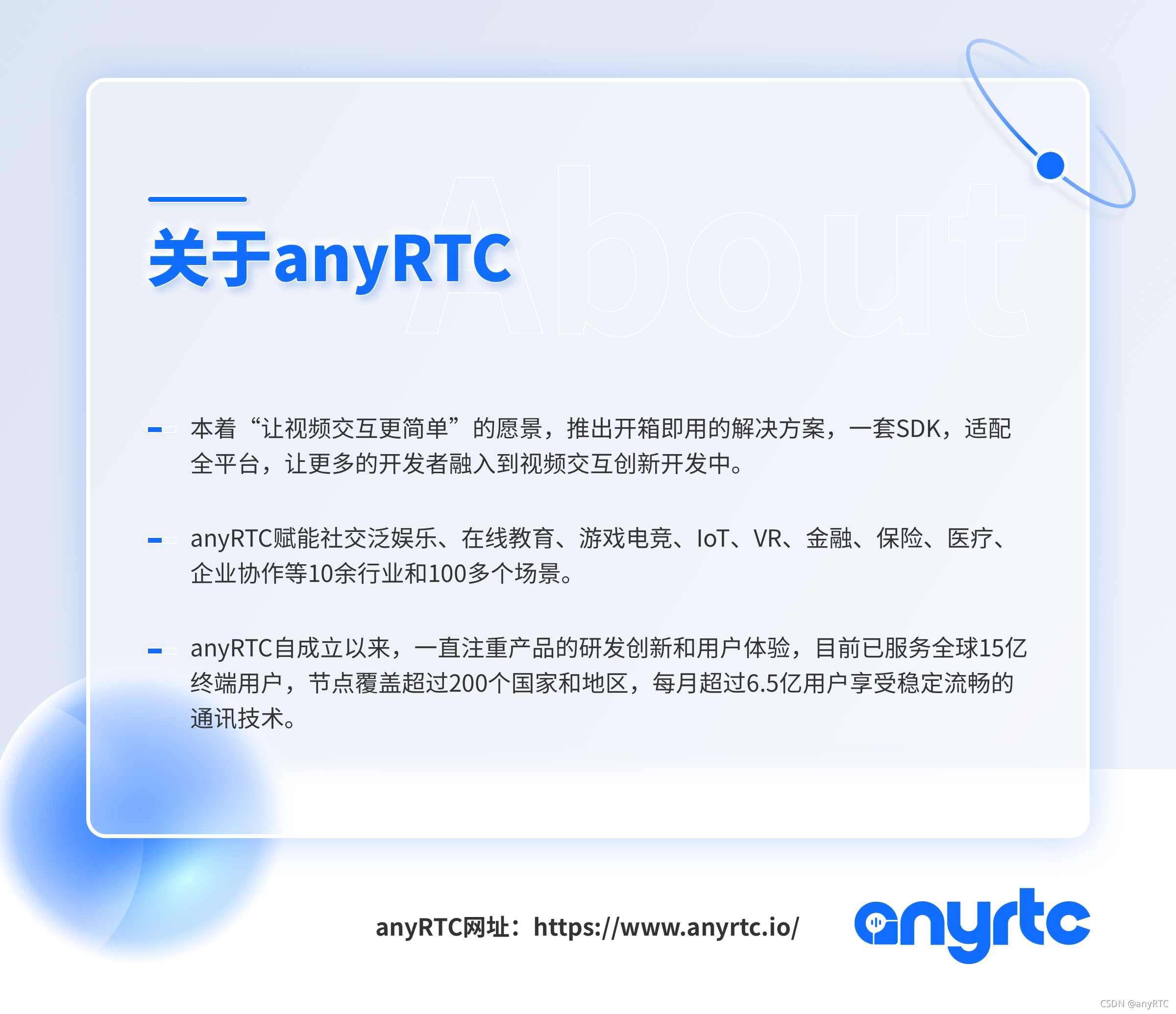